精彩的瞬间之所以珍贵
不仅是因为“瞬间”短暂
“瞬间”可遇不可求
流逝的时光之所以珍贵
不仅是因为“时光”易逝
“时光”一去不复返
抓拍模式/Video Tagging
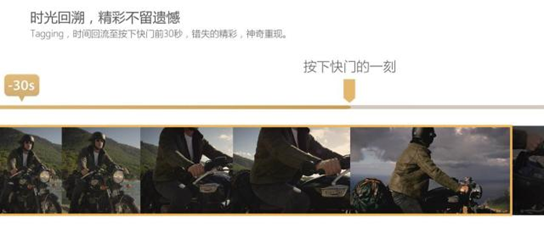
不在错过 把瞬间留住
不留遗憾 让时光回溯
拒绝拍摄大段平庸画面
有效节省相机SD卡存储空间
捕捉令人难忘的精彩瞬间
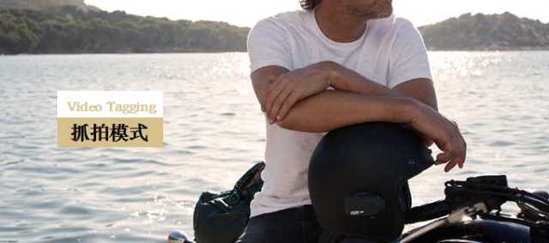
如何设置抓拍模式/Video Tagging
第一步
按下前键启动相机,然后短按相机后键切换到“设置菜单”;
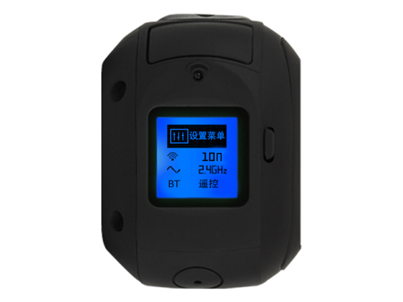
第二步
通过中键将菜单滚动到“抓拍模式”选项;
当“抓拍模式”被选中,按下后键开启(关闭)“抓拍模式”;
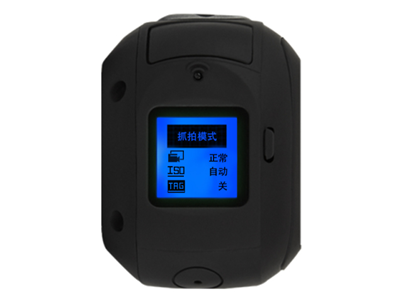
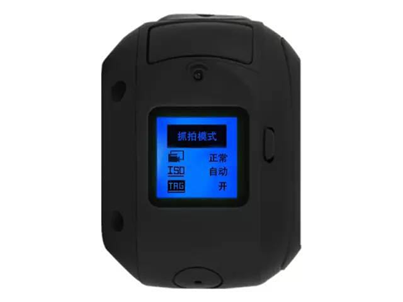
第三步
再次短按中键将菜单滚动到“抓拍时长”选项,短按后键选择抓拍时长10S/30S/1Min/2Min;
选择10秒的抓拍间隔,相机将保存抓拍前10秒,抓拍后10秒,即共计20秒的抓拍镜头;
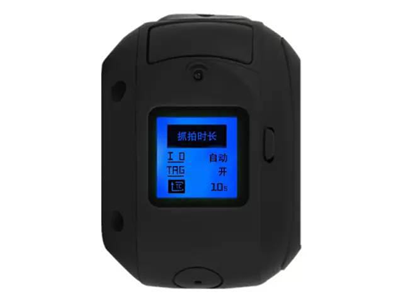
选择30秒的抓拍间隔,相机将保存抓拍前30秒,抓拍后30秒,即共计60秒的抓拍镜头;
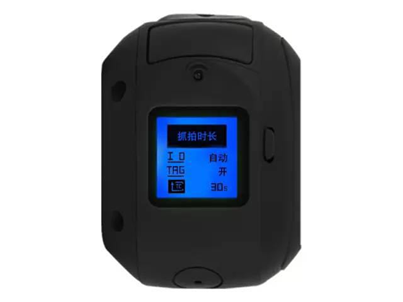
选择1分钟的抓拍间隔,相机将保存抓拍前1分钟,抓拍后1分钟,即共计2分钟的抓拍镜头;
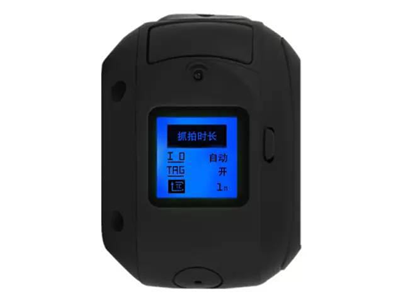
选择2分钟的抓拍间隔,相机将保存抓拍前2分钟,抓拍后2分钟,即共计4分钟的抓拍镜头。
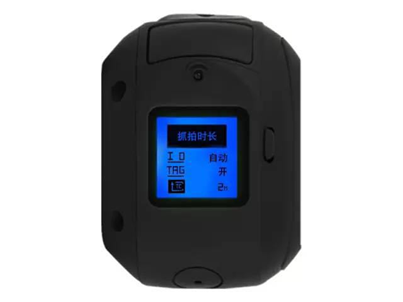
提示
长按中键可让菜单倒序滚动
“抓拍模式”下相机的“视频模式”将会被禁用,前往“设置菜单”中关闭“抓拍模式”即可恢复“视频模式”
激活“抓拍模式”功能的同时将禁用访问“车载记录仪模式”
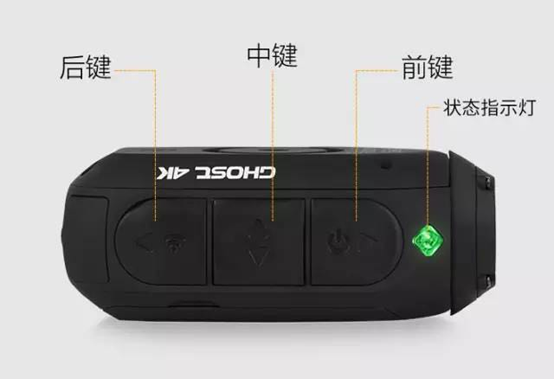
如何使用Ghost 4K抓拍模式/Video Tagging
第一步
激活“抓拍模式”,相机将开始连续录制视频,但并不会保存至SD卡中占用内存,此时相机后屏和状态指示灯将持续闪烁绿色;
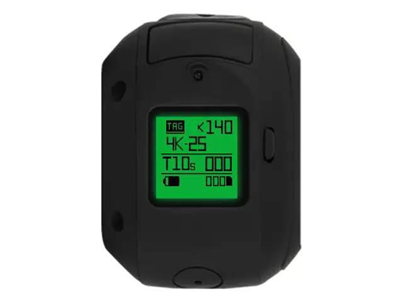
第二步
短按相机前键,进入抓拍模式,相机后屏将闪烁Tagging指令并常亮红色背光,同时相机状态指示灯将持续闪烁红色,直到完成设定时长拍摄,刚刚“抓拍”到的镜头将会被保存至相机SD卡中;
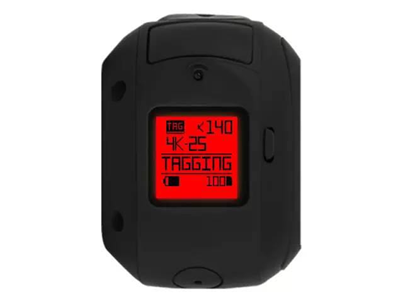
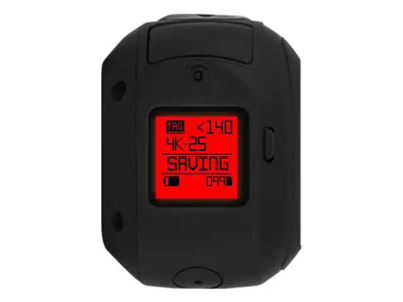
第三步
相机再次进入抓拍待命状态,后屏和状态指示灯将持续闪烁绿色;
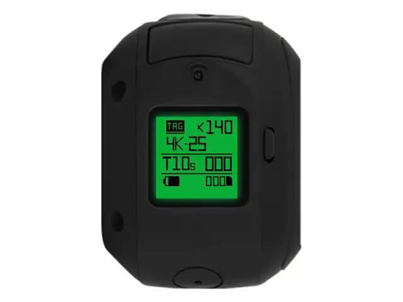
第四步
短按相机后键,即可退出“抓拍模式”;
提示:
请在启动“抓拍模式”前,设置好视频分辨率和帧率;
“抓拍模式”功能要求可用空间为4GB的 Class 10 micro SD卡,以正常运行;
请购买Class 10或等级更高的microSD/ HC/ XC卡 (最大支持128GB),首次使用前请务必将卡格式化!